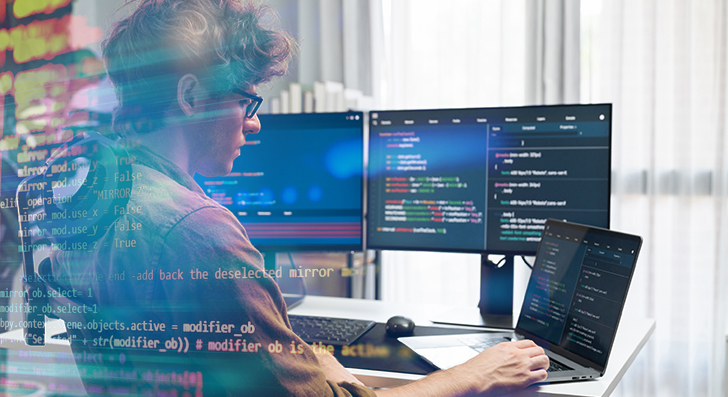
Scalability implies your software can take care of progress—much more users, additional knowledge, and a lot more site visitors—without having breaking. As being a developer, setting up with scalability in your mind will save time and tension later on. Here’s a transparent and functional manual to help you start out by Gustavo Woltmann.
Design and style for Scalability from the Start
Scalability just isn't anything you bolt on later—it ought to be portion of your system from the beginning. Lots of programs are unsuccessful whenever they increase fast mainly because the original style and design can’t deal with the additional load. As a developer, you'll want to Feel early about how your process will behave under pressure.
Start out by creating your architecture being flexible. Prevent monolithic codebases wherever all the things is tightly connected. As a substitute, use modular layout or microservices. These styles crack your app into lesser, independent areas. Each module or services can scale on its own without affecting The entire process.
Also, think of your databases from day a person. Will it require to take care of a million consumers or maybe 100? Pick the correct variety—relational or NoSQL—dependant on how your data will expand. Prepare for sharding, indexing, and backups early, Even though you don’t will need them yet.
A different crucial place is to stay away from hardcoding assumptions. Don’t write code that only functions below present-day circumstances. Think of what would transpire In the event your user base doubled tomorrow. Would your app crash? Would the database decelerate?
Use design designs that assist scaling, like concept queues or function-pushed programs. These support your application tackle more requests with out finding overloaded.
After you Establish with scalability in mind, you are not just making ready for fulfillment—you happen to be minimizing foreseeable future head aches. A effectively-prepared technique is simpler to maintain, adapt, and develop. It’s much better to prepare early than to rebuild afterwards.
Use the ideal Databases
Picking out the ideal databases is often a essential Portion of creating scalable programs. Not all databases are constructed exactly the same, and utilizing the wrong you can gradual you down or simply trigger failures as your app grows.
Start by understanding your data. Is it highly structured, like rows in a desk? If yes, a relational databases like PostgreSQL or MySQL is an efficient match. They are strong with interactions, transactions, and consistency. They also aid scaling approaches like examine replicas, indexing, and partitioning to deal with much more targeted visitors and info.
In case your data is much more flexible—like person action logs, product catalogs, or documents—look at a NoSQL selection like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing substantial volumes of unstructured or semi-structured info and will scale horizontally additional effortlessly.
Also, take into consideration your read through and publish patterns. Will you be carrying out many reads with less writes? Use caching and read replicas. Are you dealing with a major create load? Look into databases that could tackle higher produce throughput, or even occasion-based mostly details storage methods like Apache Kafka (for momentary data streams).
It’s also wise to Feel in advance. You might not want State-of-the-art scaling attributes now, but selecting a databases that supports them usually means you received’t require to change afterwards.
Use indexing to speed up queries. Prevent needless joins. Normalize or denormalize your knowledge depending on your accessibility designs. And generally observe databases performance as you improve.
To put it briefly, the proper database is dependent upon your application’s framework, pace requirements, And the way you assume it to improve. Choose time to choose correctly—it’ll help you save a lot of trouble later.
Optimize Code and Queries
Quick code is vital to scalability. As your app grows, each and every modest hold off provides up. Improperly published code or unoptimized queries can decelerate general performance and overload your process. That’s why it’s imperative that you Establish economical logic from the beginning.
Start off by composing clear, very simple code. Prevent repeating logic and remove something unnecessary. Don’t pick the most advanced Option if an easy a single operates. Keep your capabilities brief, focused, and straightforward to check. Use profiling resources to locate bottlenecks—locations exactly where your code requires much too very long to run or uses an excessive amount of memory.
Future, have a look at your database queries. These normally sluggish things down in excess of the code itself. Be certain Just about every query only asks for the info you really will need. Stay away from Find *, which fetches almost everything, and rather pick out particular fields. Use indexes to hurry up lookups. And stay away from doing too many joins, Primarily across substantial tables.
In the event you discover the exact same information currently being asked for repeatedly, use caching. Retail outlet the results briefly working with tools like Redis or Memcached and that means you don’t really have to repeat pricey functions.
Also, batch your database operations after you can. As opposed to updating a row one after the other, update them in teams. This cuts down on overhead and tends to make your application extra efficient.
Remember to examination with significant datasets. Code and queries that perform good with one hundred data could possibly crash when they have to deal with 1 million.
In a nutshell, scalable applications are speedy apps. Keep your code tight, your queries lean, and use caching when essential. These techniques aid your software keep sleek and responsive, at the same time as the load increases.
Leverage Load Balancing and Caching
As your app grows, it has to deal with far more buyers and more visitors. If every little thing goes by means of a person server, it'll swiftly become a bottleneck. That’s the place load balancing and caching can be found in. These two resources aid keep your app fast, secure, and scalable.
Load balancing spreads incoming targeted visitors throughout various servers. As opposed to a single server carrying out all of the function, the load balancer routes customers to various servers determined by availability. What this means is no solitary server gets overloaded. If a single server goes down, the load balancer can deliver traffic to the Many others. Instruments like Nginx, HAProxy, or cloud-based solutions from AWS and Google Cloud make this straightforward to build.
Caching is about storing info temporarily so it might be reused promptly. When end users request the same facts once again—like a product site or possibly a profile—you don’t should fetch it from your databases anytime. You'll be able to provide it through the cache.
There are two popular sorts of caching:
1. Server-facet caching (like Redis or Memcached) retailers details in memory for rapidly access.
two. Customer-side caching (like browser caching or CDN caching) outlets static information near the user.
Caching cuts down database load, increases speed, and will make your app additional productive.
Use caching for things which don’t modify often. And constantly ensure your cache is current when information does transform.
In brief, load balancing and caching are very simple but powerful resources. Jointly, they assist your app cope with more end users, continue to be quickly, and Get well from difficulties. If you propose to grow, you may need both of those.
Use Cloud and Container Resources
To create scalable apps, you need resources that allow your application mature effortlessly. That’s the place cloud platforms and containers are available. They offer you flexibility, lower set up time, and make scaling much smoother.
Cloud platforms like Amazon Internet Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and products and services as you need them. You don’t need to acquire hardware or guess long run potential. When traffic increases, you are able to include extra means with just some clicks or automatically utilizing auto-scaling. When visitors drops, you'll be able to scale down to save money.
These platforms also provide providers like managed databases, storage, load balancing, and safety resources. You'll be able to give attention to creating your app rather than managing infrastructure.
Containers are another essential Device. A container deals your app and everything it really should operate—code, libraries, configurations—into one particular unit. This makes it easy to maneuver your app among environments, from your notebook to your cloud, with no surprises. Docker is the most well-liked Device for this.
When your app works by using a number of containers, resources like Kubernetes help you regulate them. Kubernetes handles deployment, scaling, and Restoration. If a single component within your application crashes, it restarts it immediately.
Containers also enable it to be very easy to separate aspects of your app into solutions. You could update or scale pieces independently, that's great for effectiveness and reliability.
To put it briefly, employing cloud and container tools suggests you are able to scale speedy, deploy very easily, and Get better speedily when issues transpire. If you would like your application to develop devoid of limits, start off employing these tools early. They help save time, decrease possibility, and assist you to keep centered on creating, not correcting.
Keep track of Almost everything
If you don’t check your software, you received’t know when issues go Mistaken. Checking assists you see how your application is accomplishing, spot difficulties early, and make better choices as your application grows. It’s a critical part of developing scalable programs.
Start by tracking simple metrics like CPU utilization, memory, disk Place, and reaction time. These show you how your servers and services are carrying out. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you obtain and visualize this data.
Don’t just check your servers—check your app much too. Keep an eye on how long it will take for consumers to load webpages, how often problems transpire, and wherever they manifest. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s happening inside your code.
Set up alerts click here for important problems. For instance, In case your response time goes above a Restrict or maybe a assistance goes down, it is best to get notified instantly. This helps you fix challenges speedy, generally in advance of end users even recognize.
Monitoring is also practical after you make improvements. In case you deploy a fresh function and find out a spike in glitches or slowdowns, it is possible to roll it back before it results in true injury.
As your application grows, website traffic and knowledge enhance. Without having checking, you’ll miss out on signs of hassle until eventually it’s also late. But with the right instruments in position, you continue to be in control.
In short, checking assists you keep the app responsible and scalable. It’s not nearly recognizing failures—it’s about comprehension your method and making certain it works very well, even under pressure.
Closing Thoughts
Scalability isn’t only for huge providers. Even tiny applications want a solid foundation. By planning cautiously, optimizing correctly, and using the suitable tools, you may build apps that mature easily devoid of breaking under pressure. Start off small, Feel significant, and Construct good.